A simple language for developing full-stack web apps with less code.
Describe high-level features with Wasp DSL and write the rest of your logic in React, Node.js and Prisma.
curl -sSL http://get.wasp-lang.dev | sh
Wasp is an open source, declarative DSL for devs who want to use modern web dev stack (React
, Node.js
, Prisma
, ...)Â without writing boilerplate.
Frontend, backend and deployment - all unified with one concise language.
Zero configuration, all best practices.
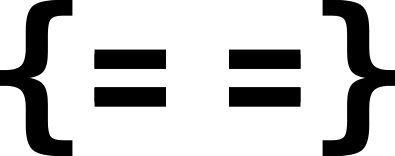
How it works
Given .wasp
+ .js
, .css
, ...
files as an input, Wasp compiler behind the scene generates the full source code of your web app - front-end, back-end and deployment.
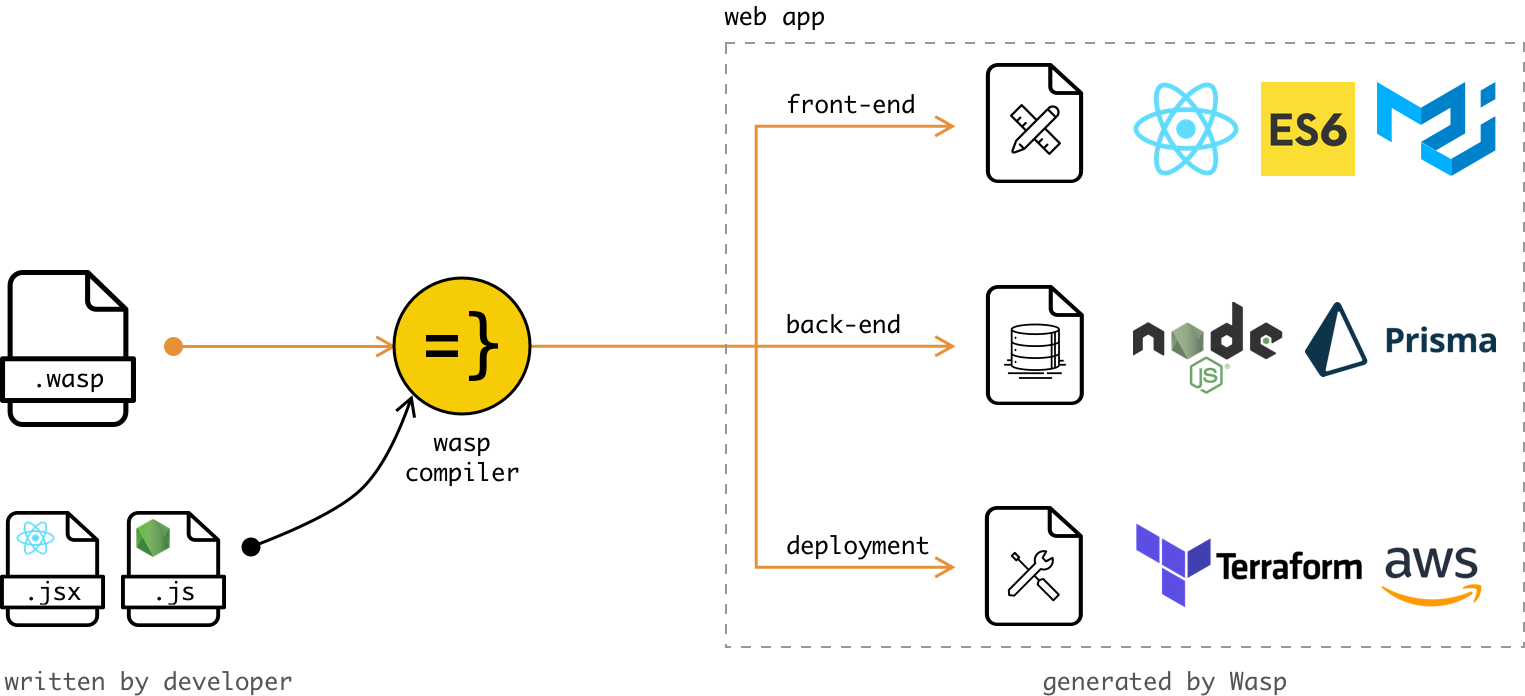
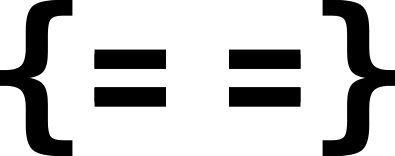
Quick start
No more endless configuration files. Create a production-ready web app with just a few lines of code - we will set you up with all the best defaults.
Speed & Power
Move fast using Wasp's declarative language, but also drop down to js/html/css...
when you require more control.
No lock-in
If Wasp becomes too limiting for you, simply eject and continue with the human-readable source code following industry best-practices.
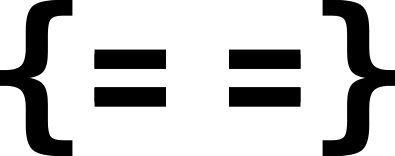
Quick to start, easy to scale
Wasp aims to be at least as flexible as the traditional web frameworks like Ruby on Rails.
Start your project quickly with the best defaults and customize and scale it as it grows.
As an example, we used Wasp to implement a copy of Medium:
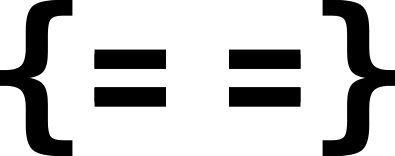
Features & Roadmap
Alpha
- full-stack auth (email & password)
- pages & routing
- blurs the line between client & server - define your server actions and queries and call them directly in your client code (RPC)!
- smart caching of server actions and queries (automatic cache invalidation)
- entity (data model) definition with Prisma.io
- ACL on frontend
- importing NPM dependencies
Coming next
- ACL on backend
- one-click deployment
- more auth methods (Google, Linkedin, ...)
- tighter integration of entities with other features
- themes and layouts
- support for explicitely defined server API
- inline JS - ability to mix JS code with Wasp code!
- Typescript support
- server-side rendering
- Visual Editor
- support for different languages on backend
- richer wasp language with better tooling
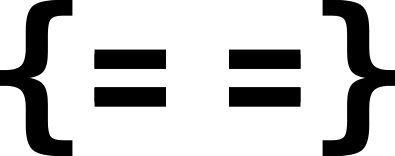
The Language
Concepts such as app, page, route, auth etc. are baked into Wasp, providing the higher level of expressiveness.
wasp start
and check it out at http://localhost:3000
!Take the tutorial
Take the Todo App tutorial and build a full-fledged Todo app in Wasp!

Stay up to date
Join our mailing list and be the first to know when we ship new features and updates!
Also, make sure to check out Wasp repo on Github and express your support by giving us a star!